8. Grey radiation modeling with climlab#
This notebook is part of The Climate Laboratory by Brian E. J. Rose, University at Albany.
1. Introducing climlab
#
About climlab#
climlab is a specialized python package for process-oriented climate modeling.
It is based on a very general concept of a model as a collection of individual,
interacting processes. climlab defines a base class called Process
, which
can contain an arbitrarily complex tree of sub-processes (each also some
sub-class of Process
). Every climate process (radiative, dynamical,
physical, turbulent, convective, chemical, etc.) can be simulated as a stand-alone
process model given appropriate input, or as a sub-process of a more complex model.
New classes of model can easily be defined and run interactively by putting together an
appropriate collection of sub-processes.
climlab is an open-source community project. The latest code can always be found on github:
Installing climlab#
If you’ve followed these instructions from the Climate Laboratory book, then you should be all set – climlab is automatically installed as part of the suite of tools used in The Climate Laboratory.
If you are maintaining your own Python installation (e.g. on a personal laptop), you can always install climlab by doing
conda install -c conda-forge climlab
%matplotlib inline
import numpy as np
import matplotlib.pyplot as plt
import xarray as xr
from numpy import cos, deg2rad, log
import climlab
2. Using climlab
to implement the two-layer leaky greenhouse model#
One of the things that climlab
is set up to do is the grey-radiation modeling we have already been discussing.
Since we already derived a [complete analytical solution to the two-layer leaky greenhouse model](Lecture06 – Elementary greenhouse models.ipynb), we will use this to validate the climlab
code.
Validation#
We want to verify that the model reproduces the observed OLR given observed temperatures, and the absorptivity that we tuned in the analytical model. The target numbers are:
Initialize a model in climlab
#
The first thing we do is create a new model.
The following example code is sparsely commented but will hopefully orient you on the basics of defining and working with a climlab Process
object.
# Test in a 2-layer atmosphere
col = climlab.GreyRadiationModel(num_lev=2)
print( col)
climlab Process of type <class 'climlab.model.column.GreyRadiationModel'>.
State variables and domain shapes:
Ts: (1,)
Tatm: (2,)
The subprocess tree:
Untitled: <class 'climlab.model.column.GreyRadiationModel'>
LW: <class 'climlab.radiation.greygas.GreyGas'>
SW: <class 'climlab.radiation.greygas.GreyGasSW'>
insolation: <class 'climlab.radiation.insolation.FixedInsolation'>
col.subprocess
AttrDict({'LW': <climlab.radiation.greygas.GreyGas object at 0x109debd00>, 'SW': <climlab.radiation.greygas.GreyGasSW object at 0x109debd60>, 'insolation': <climlab.radiation.insolation.FixedInsolation object at 0x1657336a0>})
Every item in the above dictionary is itself an instance of the climlab.Process
object:
print( col.subprocess['LW'])
climlab Process of type <class 'climlab.radiation.greygas.GreyGas'>.
State variables and domain shapes:
Ts: (1,)
Tatm: (2,)
The subprocess tree:
Untitled: <class 'climlab.radiation.greygas.GreyGas'>
The state
dictionary holds the state variables of the model. In this case, temperatures:
climlab.to_xarray(col.state)
<xarray.Dataset> Dimensions: (depth: 1, depth_bounds: 2, lev: 2, lev_bounds: 3) Coordinates: * depth (depth) float64 0.5 * depth_bounds (depth_bounds) float64 0.0 1.0 * lev (lev) float64 250.0 750.0 * lev_bounds (lev_bounds) float64 0.0 500.0 1e+03 Data variables: Ts (depth) float64 288.0 Tatm (lev) float64 200.0 278.0
- depth: 1
- depth_bounds: 2
- lev: 2
- lev_bounds: 3
- depth(depth)float640.5
- units :
- meters
array([0.5])
- depth_bounds(depth_bounds)float640.0 1.0
- units :
- meters
array([0., 1.])
- lev(lev)float64250.0 750.0
- units :
- mb
array([250., 750.])
- lev_bounds(lev_bounds)float640.0 500.0 1e+03
- units :
- mb
array([ 0., 500., 1000.])
- Ts(depth)float64288.0
array([288.])
- Tatm(lev)float64200.0 278.0
array([200., 278.])
Access these either through dictionary methods or as attributes of the model object:
print( col.state['Ts'])
print( col.Ts)
col.Ts is col.state['Ts']
[288.]
[288.]
True
Now we are assigning the “observed” temperatures to our model state:
col.Ts[:] = 288.
col.Tatm[:] = np.array([230., 275.])
climlab.to_xarray(col.state)
<xarray.Dataset> Dimensions: (depth: 1, depth_bounds: 2, lev: 2, lev_bounds: 3) Coordinates: * depth (depth) float64 0.5 * depth_bounds (depth_bounds) float64 0.0 1.0 * lev (lev) float64 250.0 750.0 * lev_bounds (lev_bounds) float64 0.0 500.0 1e+03 Data variables: Ts (depth) float64 288.0 Tatm (lev) float64 230.0 275.0
- depth: 1
- depth_bounds: 2
- lev: 2
- lev_bounds: 3
- depth(depth)float640.5
- units :
- meters
array([0.5])
- depth_bounds(depth_bounds)float640.0 1.0
- units :
- meters
array([0., 1.])
- lev(lev)float64250.0 750.0
- units :
- mb
array([250., 750.])
- lev_bounds(lev_bounds)float640.0 500.0 1e+03
- units :
- mb
array([ 0., 500., 1000.])
- Ts(depth)float64288.0
array([288.])
- Tatm(lev)float64230.0 275.0
array([230., 275.])
LW = col.subprocess['LW']
print(LW)
climlab Process of type <class 'climlab.radiation.greygas.GreyGas'>.
State variables and domain shapes:
Ts: (1,)
Tatm: (2,)
The subprocess tree:
Untitled: <class 'climlab.radiation.greygas.GreyGas'>
LW.absorptivity
Field([0.47737425, 0.47737425])
# copying the tuned value of epsilon from Lecture 6 notes
LW.absorptivity = 0.586
LW.absorptivity
Field([0.586, 0.586])
# This does all the calculations that would be performed at each time step,
# but doesn't actually update the temperatures
col.compute_diagnostics()
# Print out the dictionary
col.diagnostics
{'flux_from_sfc': Field([102.0487]),
'flux_to_sfc': array([341.3]),
'flux_to_space': array([102.0487]),
'absorbed': array([0., 0.]),
'absorbed_total': 0.0,
'emission': Field([0., 0.]),
'emission_sfc': Field([0.]),
'flux_reflected_up': array([ 0. , 0. , 102.0487]),
'insolation': Field([341.3]),
'coszen': Field([1.]),
'OLR': array([238.5247311]),
'LW_down_sfc': array([228.53426769]),
'LW_up_sfc': Field([390.10502995]),
'LW_absorbed_sfc': Field([-161.57076227]),
'LW_absorbed_atm': array([ 20.02990881, -96.98387764]),
'LW_emission': Field([ 92.98664086, 190.03779837]),
'ASR': Field([239.2513]),
'SW_absorbed_sfc': Field([239.2513]),
'SW_absorbed_atm': array([0., 0.]),
'SW_up_sfc': Field([102.0487]),
'SW_up_TOA': array([102.0487]),
'SW_down_TOA': Field([341.3]),
'planetary_albedo': Field([0.299])}
# Check OLR against our analytical solution
col.OLR
array([238.5247311])
# Like the state variables, the diagnostics can also be accessed in two different ways
col.diagnostics['OLR']
array([238.5247311])
col.state
AttrDict({'Ts': Field([288.]), 'Tatm': Field([230., 275.])})
# perform a single time step
col.step_forward()
col.state
AttrDict({'Ts': Field([289.60514636]), 'Tatm': Field([230.33784312, 273.3641795 ])})
We just stepped forward one discreet unit in time. Because we didn’t specify a timestep when we created the model, it is set to a default value:
col.timestep
86400.0
which is 1 day (expressed in seconds).
Now we will integrate the model out to equilibrium.
We could easily write a loop to call the step_forward()
method many times.
Or use a handy shortcut that allows us to specify the integration length in physical time units:
# integrate out to radiative equilibrium
col.integrate_years(2.)
Integrating for 730 steps, 730.4844 days, or 2.0 years.
Total elapsed time is 2.0014116660123062 years.
# Check for equilibrium
col.ASR - col.OLR
Field([-2.34514516e-07])
# The temperatures at radiative equilibrium
col.state
AttrDict({'Ts': Field([296.38447748]), 'Tatm': Field([233.72131685, 262.28540231])})
Compare these to the analytical solutions for radiative equilibrium with \(\epsilon = 0.58\):
So it looks like climlab
agrees with our analytical results to within 0.1 K. That’s good.
3. The observed annual, global mean temperature profile#
We want to model the OLR in a column whose temperatures match observations. As we’ve done before, we’ll calculate the global, annual mean air temperature from the NCEP Reanalysis data.
## The NOAA ESRL server is shutdown! January 2019
## This will try to read the data over the internet.
ncep_filename = 'air.mon.1981-2010.ltm.nc'
## to read over internet
ncep_url = "http://www.esrl.noaa.gov/psd/thredds/dodsC/Datasets/ncep.reanalysis.derived/pressure/"
path = ncep_url
## Open handle to data
ncep_air = xr.open_dataset( path + ncep_filename, decode_times=False )
#url = 'http://apdrc.soest.hawaii.edu:80/dods/public_data/Reanalysis_Data/NCEP/NCEP/clima/pressure/air'
#air = xr.open_dataset(url)
# The name of the vertical axis is different than the NOAA ESRL version..
#ncep_air = air.rename({'lev': 'level'})
ncep_air
<xarray.Dataset> Dimensions: (lat: 73, level: 17, lon: 144, nbnds: 2, time: 12) Coordinates: * level (level) float32 1e+03 925.0 850.0 ... 30.0 20.0 10.0 * lon (lon) float32 0.0 2.5 5.0 7.5 ... 352.5 355.0 357.5 * time (time) float64 -6.571e+05 -6.57e+05 ... -6.567e+05 * lat (lat) float32 90.0 87.5 85.0 82.5 ... -85.0 -87.5 -90.0 Dimensions without coordinates: nbnds Data variables: climatology_bounds (time, nbnds) float64 1.736e+07 1.761e+07 ... 1.762e+07 air (time, level, lat, lon) float32 ... valid_yr_count (time, level, lat, lon) float32 ... Attributes: description: Data from NCEP initialized reanalysis (4... platform: Model Conventions: COARDS not_missing_threshold_percent: minimum 3% values input to have non-missi... history: Created 2011/07/12 by doMonthLTM\nConvert... title: monthly ltm air from the NCEP Reanalysis dataset_title: NCEP-NCAR Reanalysis 1 References: http://www.psl.noaa.gov/data/gridded/data...
- lat: 73
- level: 17
- lon: 144
- nbnds: 2
- time: 12
- level(level)float321e+03 925.0 850.0 ... 20.0 10.0
- units :
- millibar
- long_name :
- Level
- positive :
- down
- GRIB_id :
- 100
- GRIB_name :
- hPa
- actual_range :
- [1000. 10.]
- axis :
- Z
array([1000., 925., 850., 700., 600., 500., 400., 300., 250., 200., 150., 100., 70., 50., 30., 20., 10.], dtype=float32)
- lon(lon)float320.0 2.5 5.0 ... 352.5 355.0 357.5
- units :
- degrees_east
- long_name :
- Longitude
- actual_range :
- [ 0. 357.5]
- standard_name :
- longitude
- axis :
- X
array([ 0. , 2.5, 5. , 7.5, 10. , 12.5, 15. , 17.5, 20. , 22.5, 25. , 27.5, 30. , 32.5, 35. , 37.5, 40. , 42.5, 45. , 47.5, 50. , 52.5, 55. , 57.5, 60. , 62.5, 65. , 67.5, 70. , 72.5, 75. , 77.5, 80. , 82.5, 85. , 87.5, 90. , 92.5, 95. , 97.5, 100. , 102.5, 105. , 107.5, 110. , 112.5, 115. , 117.5, 120. , 122.5, 125. , 127.5, 130. , 132.5, 135. , 137.5, 140. , 142.5, 145. , 147.5, 150. , 152.5, 155. , 157.5, 160. , 162.5, 165. , 167.5, 170. , 172.5, 175. , 177.5, 180. , 182.5, 185. , 187.5, 190. , 192.5, 195. , 197.5, 200. , 202.5, 205. , 207.5, 210. , 212.5, 215. , 217.5, 220. , 222.5, 225. , 227.5, 230. , 232.5, 235. , 237.5, 240. , 242.5, 245. , 247.5, 250. , 252.5, 255. , 257.5, 260. , 262.5, 265. , 267.5, 270. , 272.5, 275. , 277.5, 280. , 282.5, 285. , 287.5, 290. , 292.5, 295. , 297.5, 300. , 302.5, 305. , 307.5, 310. , 312.5, 315. , 317.5, 320. , 322.5, 325. , 327.5, 330. , 332.5, 335. , 337.5, 340. , 342.5, 345. , 347.5, 350. , 352.5, 355. , 357.5], dtype=float32)
- time(time)float64-6.571e+05 -6.57e+05 ... -6.567e+05
- long_name :
- Time
- delta_t :
- 0000-01-00 00:00:00
- avg_period :
- 0030-00-00 00:00:00
- prev_avg_period :
- 0000-01-00 00:00:00
- standard_name :
- time
- axis :
- T
- climatology :
- climatology_bounds
- climo_period :
- 1981/01/01 - 2010/12/31
- interpreted_actual_range :
- 0001/01/01 00:00:00 - 0001/12/01 00:00:00
- units :
- days since 1800-01-01 00:00:0.0
- actual_range :
- [-657073. -656739.]
array([-657073., -657042., -657014., -656983., -656953., -656922., -656892., -656861., -656830., -656800., -656769., -656739.])
- lat(lat)float3290.0 87.5 85.0 ... -87.5 -90.0
- units :
- degrees_north
- actual_range :
- [ 90. -90.]
- long_name :
- Latitude
- standard_name :
- latitude
- axis :
- Y
array([ 90. , 87.5, 85. , 82.5, 80. , 77.5, 75. , 72.5, 70. , 67.5, 65. , 62.5, 60. , 57.5, 55. , 52.5, 50. , 47.5, 45. , 42.5, 40. , 37.5, 35. , 32.5, 30. , 27.5, 25. , 22.5, 20. , 17.5, 15. , 12.5, 10. , 7.5, 5. , 2.5, 0. , -2.5, -5. , -7.5, -10. , -12.5, -15. , -17.5, -20. , -22.5, -25. , -27.5, -30. , -32.5, -35. , -37.5, -40. , -42.5, -45. , -47.5, -50. , -52.5, -55. , -57.5, -60. , -62.5, -65. , -67.5, -70. , -72.5, -75. , -77.5, -80. , -82.5, -85. , -87.5, -90. ], dtype=float32)
- climatology_bounds(time, nbnds)float64...
- long_name :
- Climate Time Boundaries
- units :
- hours since 1-1-1 00:00:0.0
array([[17356368., 17610576.], [17357112., 17611320.], [17357784., 17611992.], [17358528., 17612736.], [17359248., 17613456.], [17359992., 17614200.], [17360712., 17614920.], [17361456., 17615664.], [17362200., 17616408.], [17362920., 17617128.], [17363664., 17617872.], [17364384., 17618592.]])
- air(time, level, lat, lon)float32...
- long_name :
- Monthly Long Term Mean of Air temperature
- units :
- degC
- precision :
- 2
- var_desc :
- Air Temperature
- level_desc :
- Multiple levels
- statistic :
- Long Term Mean
- parent_stat :
- Mean
- valid_range :
- [-200. 300.]
- actual_range :
- [-89.722336 41.616005]
- dataset :
- NCEP Reanalysis Derived Products
- _ChunkSizes :
- [ 1 1 73 144]
[2144448 values with dtype=float32]
- valid_yr_count(time, level, lat, lon)float32...
- long_name :
- count of non-missing values used in mean
- _ChunkSizes :
- [ 1 1 73 144]
[2144448 values with dtype=float32]
- description :
- Data from NCEP initialized reanalysis (4x/day). These are interpolated to pressure surfaces from model (sigma) surfaces.
- platform :
- Model
- Conventions :
- COARDS
- not_missing_threshold_percent :
- minimum 3% values input to have non-missing output value
- history :
- Created 2011/07/12 by doMonthLTM Converted to chunked, deflated non-packed NetCDF4 2014/09
- title :
- monthly ltm air from the NCEP Reanalysis
- dataset_title :
- NCEP-NCAR Reanalysis 1
- References :
- http://www.psl.noaa.gov/data/gridded/data.ncep.reanalysis.derived.html
# Take global, annual average and convert to Kelvin
weight = cos(deg2rad(ncep_air.lat)) / cos(deg2rad(ncep_air.lat)).mean(dim='lat')
Tglobal = (ncep_air.air * weight).mean(dim=('lat','lon','time'))
Tglobal
<xarray.DataArray (level: 17)> array([ 15.179084 , 11.207003 , 7.8383274 , 0.21994135, -6.4483433 , -14.888848 , -25.570469 , -39.36969 , -46.797905 , -53.652245 , -60.56356 , -67.006065 , -65.53293 , -61.48664 , -55.853584 , -51.593952 , -43.21999 ], dtype=float32) Coordinates: * level (level) float32 1e+03 925.0 850.0 700.0 ... 50.0 30.0 20.0 10.0
- level: 17
- 15.18 11.21 7.838 0.2199 -6.448 ... -65.53 -61.49 -55.85 -51.59 -43.22
array([ 15.179084 , 11.207003 , 7.8383274 , 0.21994135, -6.4483433 , -14.888848 , -25.570469 , -39.36969 , -46.797905 , -53.652245 , -60.56356 , -67.006065 , -65.53293 , -61.48664 , -55.853584 , -51.593952 , -43.21999 ], dtype=float32)
- level(level)float321e+03 925.0 850.0 ... 20.0 10.0
- units :
- millibar
- long_name :
- Level
- positive :
- down
- GRIB_id :
- 100
- GRIB_name :
- hPa
- actual_range :
- [1000. 10.]
- axis :
- Z
array([1000., 925., 850., 700., 600., 500., 400., 300., 250., 200., 150., 100., 70., 50., 30., 20., 10.], dtype=float32)
We’re going to convert this to degrees Kelvin, using a handy list of pre-defined constants in climlab.constants
climlab.constants.tempCtoK
273.15
Tglobal += climlab.constants.tempCtoK
Tglobal
<xarray.DataArray (level: 17)> array([288.32907, 284.357 , 280.9883 , 273.36993, 266.70166, 258.26114, 247.57953, 233.7803 , 226.35208, 219.49774, 212.58643, 206.14392, 207.61707, 211.66336, 217.29642, 221.55605, 229.93001], dtype=float32) Coordinates: * level (level) float32 1e+03 925.0 850.0 700.0 ... 50.0 30.0 20.0 10.0
- level: 17
- 288.3 284.4 281.0 273.4 266.7 258.3 ... 207.6 211.7 217.3 221.6 229.9
array([288.32907, 284.357 , 280.9883 , 273.36993, 266.70166, 258.26114, 247.57953, 233.7803 , 226.35208, 219.49774, 212.58643, 206.14392, 207.61707, 211.66336, 217.29642, 221.55605, 229.93001], dtype=float32)
- level(level)float321e+03 925.0 850.0 ... 20.0 10.0
- units :
- millibar
- long_name :
- Level
- positive :
- down
- GRIB_id :
- 100
- GRIB_name :
- hPa
- actual_range :
- [1000. 10.]
- axis :
- Z
array([1000., 925., 850., 700., 600., 500., 400., 300., 250., 200., 150., 100., 70., 50., 30., 20., 10.], dtype=float32)
# A handy re-usable routine for making a plot of the temperature profiles
# We will plot temperatures with respect to log(pressure) to get a height-like coordinate
def zstar(lev):
return -np.log(lev / climlab.constants.ps)
def plot_soundings(result_list, name_list, plot_obs=True, fixed_range=True):
color_cycle=['r', 'g', 'b', 'y']
# col is either a column model object or a list of column model objects
#if isinstance(state_list, climlab.Process):
# # make a list with a single item
# collist = [collist]
fig, ax = plt.subplots(figsize=(9,9))
if plot_obs:
ax.plot(Tglobal, zstar(Tglobal.level), color='k', label='Observed')
for i, state in enumerate(result_list):
Tatm = state['Tatm']
lev = Tatm.domain.axes['lev'].points
Ts = state['Ts']
ax.plot(Tatm, zstar(lev), color=color_cycle[i], label=name_list[i])
ax.plot(Ts, 0, 'o', markersize=12, color=color_cycle[i])
#ax.invert_yaxis()
yticks = np.array([1000., 750., 500., 250., 100., 50., 20., 10., 5.])
ax.set_yticks(-np.log(yticks/1000.))
ax.set_yticklabels(yticks)
ax.set_xlabel('Temperature (K)', fontsize=14)
ax.set_ylabel('Pressure (hPa)', fontsize=14)
ax.grid()
ax.legend()
if fixed_range:
ax.set_xlim([200, 300])
ax.set_ylim(zstar(np.array([1000., 5.])))
#ax2 = ax.twinx()
return ax
plot_soundings([],[] );
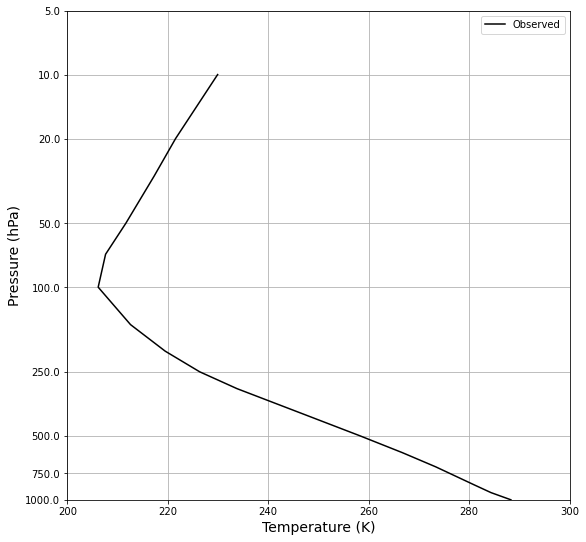
4. A 30-layer model using the observed temperatures#
# initialize a grey radiation model with 30 levels
col = climlab.GreyRadiationModel()
print(col)
climlab Process of type <class 'climlab.model.column.GreyRadiationModel'>.
State variables and domain shapes:
Ts: (1,)
Tatm: (30,)
The subprocess tree:
Untitled: <class 'climlab.model.column.GreyRadiationModel'>
LW: <class 'climlab.radiation.greygas.GreyGas'>
SW: <class 'climlab.radiation.greygas.GreyGasSW'>
insolation: <class 'climlab.radiation.insolation.FixedInsolation'>
col.lev
array([ 16.66666667, 50. , 83.33333333, 116.66666667,
150. , 183.33333333, 216.66666667, 250. ,
283.33333333, 316.66666667, 350. , 383.33333333,
416.66666667, 450. , 483.33333333, 516.66666667,
550. , 583.33333333, 616.66666667, 650. ,
683.33333333, 716.66666667, 750. , 783.33333333,
816.66666667, 850. , 883.33333333, 916.66666667,
950. , 983.33333333])
col.lev_bounds
array([ 0. , 33.33333333, 66.66666667, 100. ,
133.33333333, 166.66666667, 200. , 233.33333333,
266.66666667, 300. , 333.33333333, 366.66666667,
400. , 433.33333333, 466.66666667, 500. ,
533.33333333, 566.66666667, 600. , 633.33333333,
666.66666667, 700. , 733.33333333, 766.66666667,
800. , 833.33333333, 866.66666667, 900. ,
933.33333333, 966.66666667, 1000. ])
# interpolate to 30 evenly spaced pressure levels
lev = col.lev
Tinterp = np.interp(lev, np.flipud(Tglobal.level), np.flipud(Tglobal))
Tinterp
# Need to 'flipud' because the interpolation routine
# needs the pressure data to be in increasing order
array([224.34736633, 211.6633606 , 206.96233453, 208.29142253,
212.58642578, 217.19396973, 221.78252157, 226.3520813 ,
231.30422974, 236.08017476, 240.67991638, 245.279658 ,
249.35979716, 252.92033386, 256.48087056, 259.66789246,
262.48139954, 265.29490662, 267.81303914, 270.03579712,
272.25855509, 274.21642049, 275.90939331, 277.60236613,
279.29533895, 280.98831177, 282.48550415, 283.98269653,
285.6810201 , 287.4463874 ])
# Initialize model with observed temperatures
col.Ts[:] = Tglobal[0]
col.Tatm[:] = Tinterp
# This should look just like the observations
result_list = [col.state]
name_list = ['Observed, interpolated']
plot_soundings(result_list, name_list);
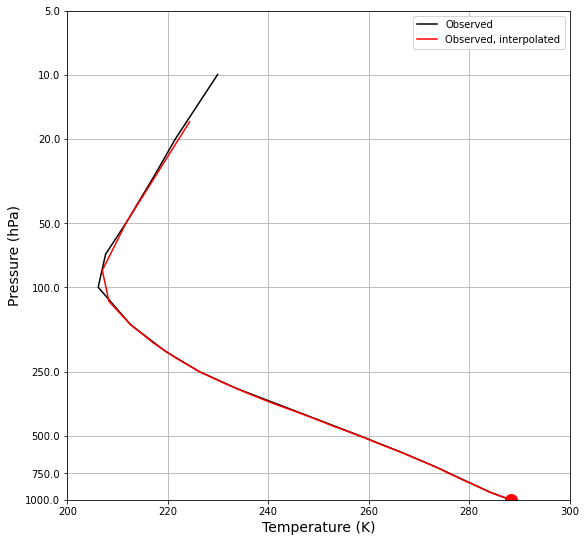
Tune absorptivity to get observed OLR#
col.compute_diagnostics()
col.OLR
array([263.15000104])
# Need to tune absorptivity to get OLR = 238.5
epsarray = np.linspace(0.01, 0.1, 100)
OLRarray = np.zeros_like(epsarray)
for i in range(epsarray.size):
col.subprocess['LW'].absorptivity = epsarray[i]
col.compute_diagnostics()
OLRarray[i] = col.OLR
plt.plot(epsarray, OLRarray)
plt.grid()
plt.xlabel('epsilon')
plt.ylabel('OLR')
Text(0, 0.5, 'OLR')
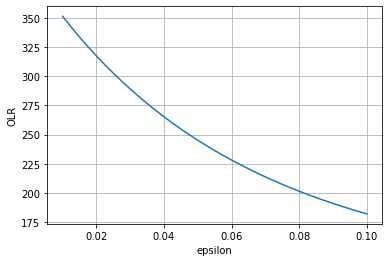
The necessary value seems to lie near 0.055 or so.
We can be more precise with a numerical root-finder.
def OLRanom(eps):
col.subprocess['LW'].absorptivity = eps
col.compute_diagnostics()
return col.OLR - 238.5
# Use numerical root-finding to get the equilibria
from scipy.optimize import brentq
# brentq is a root-finding function
# Need to give it a function and two end-points
# It will look for a zero of the function between those end-points
eps = brentq(OLRanom, 0.01, 0.1)
print( eps)
0.05369073166506245
col.subprocess.LW.absorptivity = eps
col.subprocess.LW.absorptivity
Field([0.05369073, 0.05369073, 0.05369073, 0.05369073, 0.05369073,
0.05369073, 0.05369073, 0.05369073, 0.05369073, 0.05369073,
0.05369073, 0.05369073, 0.05369073, 0.05369073, 0.05369073,
0.05369073, 0.05369073, 0.05369073, 0.05369073, 0.05369073,
0.05369073, 0.05369073, 0.05369073, 0.05369073, 0.05369073,
0.05369073, 0.05369073, 0.05369073, 0.05369073, 0.05369073])
col.compute_diagnostics()
col.OLR
array([238.5])
5. Radiative forcing in the 30-layer model#
Let’s compute radiative forcing for a 2% increase in absorptivity.
# clone our model using a built-in climlab function
col2 = climlab.process_like(col)
print(col2)
climlab Process of type <class 'climlab.model.column.GreyRadiationModel'>.
State variables and domain shapes:
Ts: (1,)
Tatm: (30,)
The subprocess tree:
Untitled: <class 'climlab.model.column.GreyRadiationModel'>
LW: <class 'climlab.radiation.greygas.GreyGas'>
SW: <class 'climlab.radiation.greygas.GreyGasSW'>
insolation: <class 'climlab.radiation.insolation.FixedInsolation'>
col2.subprocess['LW'].absorptivity *= 1.02
col2.subprocess['LW'].absorptivity
Field([0.05476455, 0.05476455, 0.05476455, 0.05476455, 0.05476455,
0.05476455, 0.05476455, 0.05476455, 0.05476455, 0.05476455,
0.05476455, 0.05476455, 0.05476455, 0.05476455, 0.05476455,
0.05476455, 0.05476455, 0.05476455, 0.05476455, 0.05476455,
0.05476455, 0.05476455, 0.05476455, 0.05476455, 0.05476455,
0.05476455, 0.05476455, 0.05476455, 0.05476455, 0.05476455])
# Radiative forcing by definition is the change in TOA radiative flux,
# HOLDING THE TEMPERATURES FIXED.
col2.Ts - col.Ts
Field([0.])
col2.Tatm - col.Tatm
Field([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.,
0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
col2.compute_diagnostics()
col2.OLR
array([236.65384137])
The OLR decreased after we added the extra absorbers, as we expect. Now we can calculate the Radiative Forcing:
RF = -(col2.OLR - col.OLR)
print( 'The radiative forcing is %.2f W/m2.' %RF)
The radiative forcing is 1.85 W/m2.
6. Radiative equilibrium in the 30-layer model#
re = climlab.process_like(col)
# To get to equilibrium, we just time-step the model forward long enough
re.integrate_years(1.)
Integrating for 365 steps, 365.2422 days, or 1.0 years.
Total elapsed time is 0.9993368783782377 years.
# Check for energy balance
print( 'The net downward radiative flux at TOA is %.4f W/m2.' %(re.ASR - re.OLR))
The net downward radiative flux at TOA is -0.0015 W/m2.
result_list.append(re.state)
name_list.append('Radiative equilibrium (grey gas)')
plot_soundings(result_list, name_list)
<AxesSubplot:xlabel='Temperature (K)', ylabel='Pressure (hPa)'>
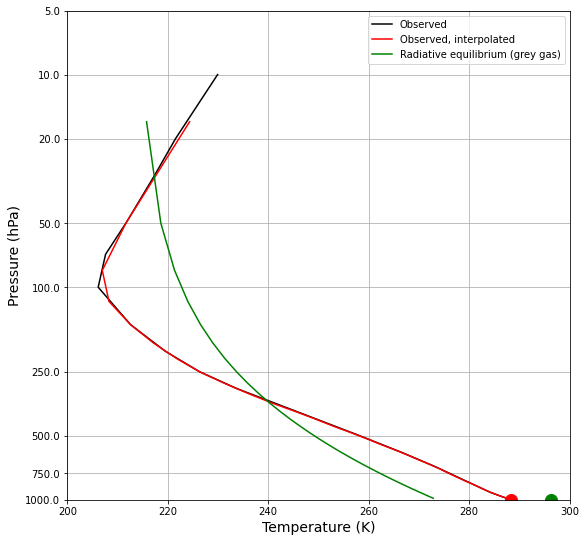
Some properties of the radiative equilibrium temperature profile:
The surface is warmer than observed.
The lower troposphere is colder than observed.
Very cold air is sitting immediately above the warm surface.
There is no tropopause, no stratosphere.
7. Radiative-Convective Equilibrium in the 30-layer model#
We recognize that the large drop in temperature just above the surface is unphysical. Parcels of air in direct contact with the ground will be warmed by mechansisms other than radiative transfer.
These warm air parcels will then become buoyant, and will convect upward, mixing their heat content with the environment.
We parameterize the statistical effects of this mixing through a convective adjustment.
At each timestep, our model checks for any locations at which the lapse rate exceeds some threshold. Unstable layers are removed through an energy-conserving mixing formula.
This process is assumed to be fast relative to radiative heating. In the model, it is instantaneous.
Add the convective adjustment as an additional subprocess#
# Here is the existing model
print(re)
climlab Process of type <class 'climlab.model.column.GreyRadiationModel'>.
State variables and domain shapes:
Ts: (1,)
Tatm: (30,)
The subprocess tree:
Untitled: <class 'climlab.model.column.GreyRadiationModel'>
LW: <class 'climlab.radiation.greygas.GreyGas'>
SW: <class 'climlab.radiation.greygas.GreyGasSW'>
insolation: <class 'climlab.radiation.insolation.FixedInsolation'>
# First we make a new clone
rce = climlab.process_like(re)
# Then create a new ConvectiveAdjustment process
conv = climlab.convection.ConvectiveAdjustment(state=rce.state,
adj_lapse_rate=6.)
# And add it to our model
rce.add_subprocess('Convective Adjustment', conv)
print(rce)
climlab Process of type <class 'climlab.model.column.GreyRadiationModel'>.
State variables and domain shapes:
Ts: (1,)
Tatm: (30,)
The subprocess tree:
Untitled: <class 'climlab.model.column.GreyRadiationModel'>
LW: <class 'climlab.radiation.greygas.GreyGas'>
SW: <class 'climlab.radiation.greygas.GreyGasSW'>
insolation: <class 'climlab.radiation.insolation.FixedInsolation'>
Convective Adjustment: <class 'climlab.convection.convadj.ConvectiveAdjustment'>
This model is exactly like our previous models, except for one additional subprocess called Convective Adjustment
.
We passed a parameter adj_lapse_rate
(in K / km) that sets the neutrally stable lapse rate – in this case, 6 K / km.
This number is chosed to very loosely represent the net effect of moist convection.
# Run out to equilibrium
rce.integrate_years(1.)
Integrating for 365 steps, 365.2422 days, or 1.0 years.
Total elapsed time is 1.9986737567564754 years.
# Check for energy balance
rce.ASR - rce.OLR
Field([0.0007796])
result_list.append(rce.state)
name_list.append('Radiatve-Convective equilibrium (grey gas)')
plot_soundings(result_list, name_list)
<AxesSubplot:xlabel='Temperature (K)', ylabel='Pressure (hPa)'>
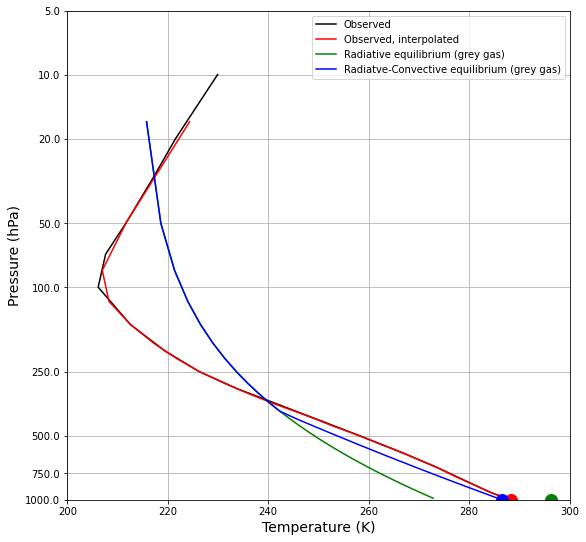
Introducing convective adjustment into the model cools the surface quite a bit (compared to Radiative Equilibrium, in green here) – and warms the lower troposphere. It gives us a MUCH better fit to observations.
But of course we still have no stratosphere.
8. Putting stratospheric ozone in the grey-gas model#
Our model has no equivalent of the stratosphere, where temperature increases with height. That’s because our model has been completely transparent to shortwave radiation up until now.
We can load the observed ozone climatology from the input files for the CESM model:
datapath = "http://thredds.atmos.albany.edu:8080/thredds/dodsC/CESMA/"
ozone = xr.open_dataset( datapath + "som_input/ozone_1.9x2.5_L26_2000clim_c091112.nc")
ozone
<xarray.Dataset> Dimensions: (ilev: 27, lat: 96, lev: 26, lon: 144, slat: 95, slon: 144, time: 12) Coordinates: * ilev (ilev) float64 2.194 4.895 9.882 18.05 ... 903.3 956.0 985.1 1e+03 * lat (lat) float64 -90.0 -88.11 -86.21 -84.32 ... 84.32 86.21 88.11 90.0 * lev (lev) float64 3.545 7.389 13.97 23.94 ... 867.2 929.6 970.6 992.6 * lon (lon) float64 0.0 2.5 5.0 7.5 10.0 ... 350.0 352.5 355.0 357.5 * slat (slat) float64 -89.05 -87.16 -85.26 -83.37 ... 85.26 87.16 89.05 * slon (slon) float64 -1.25 1.25 3.75 6.25 ... 348.8 351.2 353.8 356.2 * time (time) object 2000-01-15 00:00:00 ... 2000-12-15 00:00:00 Data variables: P0 float64 1e+05 date (time) int32 20000115 20000215 20000315 ... 20001115 20001215 datesec (time) int32 0 0 0 0 0 0 0 0 0 0 0 0 gw (lat) float64 0.0001367 0.001093 0.002185 ... 0.001093 0.0001367 hyai (ilev) float64 0.002194 0.004895 0.009882 ... 0.002521 0.0 0.0 hyam (lev) float64 0.003545 0.007389 0.01397 ... 0.004803 0.001261 0.0 hybi (ilev) float64 0.0 0.0 0.0 0.0 0.0 ... 0.8962 0.9535 0.9851 1.0 hybm (lev) float64 0.0 0.0 0.0 0.0 0.0 ... 0.8569 0.9248 0.9693 0.9926 w_stag (slat) float64 0.0005467 0.00164 0.002731 ... 0.00164 0.0005467 O3 (time, lev, lat, lon) float32 ... PS (time, lat, lon) float32 ... Attributes: (12/14) Conventions: CF-1.0 source: CAM case: ar5_cam_1850-2000_03 title: logname: lamar host: be0207en.ucar.ed ... ... initial_file: /ptmp/lamar/cam-inputs/ar5_cam_1850_06.c... topography_file: /fs/cgd/csm/inputdata/atm/cam/topo/USGS-... history: Wed Jan 5 14:56:28 2011: ncks -d time,1... nco_openmp_thread_number: 1 NCO: 4.0.5 DODS_EXTRA.Unlimited_Dimension: time
- ilev: 27
- lat: 96
- lev: 26
- lon: 144
- slat: 95
- slon: 144
- time: 12
- ilev(ilev)float642.194 4.895 9.882 ... 985.1 1e+03
- long_name :
- hybrid level at interfaces (1000*(A+B))
- units :
- level
- positive :
- down
- standard_name :
- atmosphere_hybrid_sigma_pressure_coordinate
- formula_terms :
- a: hyai b: hybi p0: P0 ps: PS
array([ 2.194067, 4.895209, 9.882418, 18.05201 , 29.83724 , 44.62334 , 61.60587 , 78.51243 , 92.3658 , 108.66359 , 127.83708 , 150.39371 , 176.93043 , 208.14944 , 244.87709 , 288.08522 , 338.91731 , 398.71865 , 469.0718 , 551.83871 , 649.20969 , 744.38289 , 831.02123 , 903.30029 , 955.99746 , 985.1122 , 1000. ])
- lat(lat)float64-90.0 -88.11 -86.21 ... 88.11 90.0
- long_name :
- latitude
- units :
- degrees_north
array([-90. , -88.105263, -86.210526, -84.315789, -82.421053, -80.526316, -78.631579, -76.736842, -74.842105, -72.947368, -71.052632, -69.157895, -67.263158, -65.368421, -63.473684, -61.578947, -59.684211, -57.789474, -55.894737, -54. , -52.105263, -50.210526, -48.315789, -46.421053, -44.526316, -42.631579, -40.736842, -38.842105, -36.947368, -35.052632, -33.157895, -31.263158, -29.368421, -27.473684, -25.578947, -23.684211, -21.789474, -19.894737, -18. , -16.105263, -14.210526, -12.315789, -10.421053, -8.526316, -6.631579, -4.736842, -2.842105, -0.947368, 0.947368, 2.842105, 4.736842, 6.631579, 8.526316, 10.421053, 12.315789, 14.210526, 16.105263, 18. , 19.894737, 21.789474, 23.684211, 25.578947, 27.473684, 29.368421, 31.263158, 33.157895, 35.052632, 36.947368, 38.842105, 40.736842, 42.631579, 44.526316, 46.421053, 48.315789, 50.210526, 52.105263, 54. , 55.894737, 57.789474, 59.684211, 61.578947, 63.473684, 65.368421, 67.263158, 69.157895, 71.052632, 72.947368, 74.842105, 76.736842, 78.631579, 80.526316, 82.421053, 84.315789, 86.210526, 88.105263, 90. ])
- lev(lev)float643.545 7.389 13.97 ... 970.6 992.6
- long_name :
- hybrid level at midpoints (1000*(A+B))
- units :
- level
- positive :
- down
- standard_name :
- atmosphere_hybrid_sigma_pressure_coordinate
- formula_terms :
- a: hyam b: hybm p0: P0 ps: PS
array([ 3.544638, 7.388814, 13.967214, 23.944625, 37.23029 , 53.114605, 70.05915 , 85.439115, 100.514695, 118.250335, 139.115395, 163.66207 , 192.539935, 226.513265, 266.481155, 313.501265, 368.81798 , 433.895225, 510.455255, 600.5242 , 696.79629 , 787.70206 , 867.16076 , 929.648875, 970.55483 , 992.5561 ])
- lon(lon)float640.0 2.5 5.0 ... 352.5 355.0 357.5
- long_name :
- longitude
- units :
- degrees_east
array([ 0. , 2.5, 5. , 7.5, 10. , 12.5, 15. , 17.5, 20. , 22.5, 25. , 27.5, 30. , 32.5, 35. , 37.5, 40. , 42.5, 45. , 47.5, 50. , 52.5, 55. , 57.5, 60. , 62.5, 65. , 67.5, 70. , 72.5, 75. , 77.5, 80. , 82.5, 85. , 87.5, 90. , 92.5, 95. , 97.5, 100. , 102.5, 105. , 107.5, 110. , 112.5, 115. , 117.5, 120. , 122.5, 125. , 127.5, 130. , 132.5, 135. , 137.5, 140. , 142.5, 145. , 147.5, 150. , 152.5, 155. , 157.5, 160. , 162.5, 165. , 167.5, 170. , 172.5, 175. , 177.5, 180. , 182.5, 185. , 187.5, 190. , 192.5, 195. , 197.5, 200. , 202.5, 205. , 207.5, 210. , 212.5, 215. , 217.5, 220. , 222.5, 225. , 227.5, 230. , 232.5, 235. , 237.5, 240. , 242.5, 245. , 247.5, 250. , 252.5, 255. , 257.5, 260. , 262.5, 265. , 267.5, 270. , 272.5, 275. , 277.5, 280. , 282.5, 285. , 287.5, 290. , 292.5, 295. , 297.5, 300. , 302.5, 305. , 307.5, 310. , 312.5, 315. , 317.5, 320. , 322.5, 325. , 327.5, 330. , 332.5, 335. , 337.5, 340. , 342.5, 345. , 347.5, 350. , 352.5, 355. , 357.5])
- slat(slat)float64-89.05 -87.16 ... 87.16 89.05
- long_name :
- staggered latitude
- units :
- degrees_north
array([-89.052632, -87.157895, -85.263158, -83.368421, -81.473684, -79.578947, -77.684211, -75.789474, -73.894737, -72. , -70.105263, -68.210526, -66.315789, -64.421053, -62.526316, -60.631579, -58.736842, -56.842105, -54.947368, -53.052632, -51.157895, -49.263158, -47.368421, -45.473684, -43.578947, -41.684211, -39.789474, -37.894737, -36. , -34.105263, -32.210526, -30.315789, -28.421053, -26.526316, -24.631579, -22.736842, -20.842105, -18.947368, -17.052632, -15.157895, -13.263158, -11.368421, -9.473684, -7.578947, -5.684211, -3.789474, -1.894737, 0. , 1.894737, 3.789474, 5.684211, 7.578947, 9.473684, 11.368421, 13.263158, 15.157895, 17.052632, 18.947368, 20.842105, 22.736842, 24.631579, 26.526316, 28.421053, 30.315789, 32.210526, 34.105263, 36. , 37.894737, 39.789474, 41.684211, 43.578947, 45.473684, 47.368421, 49.263158, 51.157895, 53.052632, 54.947368, 56.842105, 58.736842, 60.631579, 62.526316, 64.421053, 66.315789, 68.210526, 70.105263, 72. , 73.894737, 75.789474, 77.684211, 79.578947, 81.473684, 83.368421, 85.263158, 87.157895, 89.052632])
- slon(slon)float64-1.25 1.25 3.75 ... 353.8 356.2
- long_name :
- staggered longitude
- units :
- degrees_east
array([ -1.25, 1.25, 3.75, 6.25, 8.75, 11.25, 13.75, 16.25, 18.75, 21.25, 23.75, 26.25, 28.75, 31.25, 33.75, 36.25, 38.75, 41.25, 43.75, 46.25, 48.75, 51.25, 53.75, 56.25, 58.75, 61.25, 63.75, 66.25, 68.75, 71.25, 73.75, 76.25, 78.75, 81.25, 83.75, 86.25, 88.75, 91.25, 93.75, 96.25, 98.75, 101.25, 103.75, 106.25, 108.75, 111.25, 113.75, 116.25, 118.75, 121.25, 123.75, 126.25, 128.75, 131.25, 133.75, 136.25, 138.75, 141.25, 143.75, 146.25, 148.75, 151.25, 153.75, 156.25, 158.75, 161.25, 163.75, 166.25, 168.75, 171.25, 173.75, 176.25, 178.75, 181.25, 183.75, 186.25, 188.75, 191.25, 193.75, 196.25, 198.75, 201.25, 203.75, 206.25, 208.75, 211.25, 213.75, 216.25, 218.75, 221.25, 223.75, 226.25, 228.75, 231.25, 233.75, 236.25, 238.75, 241.25, 243.75, 246.25, 248.75, 251.25, 253.75, 256.25, 258.75, 261.25, 263.75, 266.25, 268.75, 271.25, 273.75, 276.25, 278.75, 281.25, 283.75, 286.25, 288.75, 291.25, 293.75, 296.25, 298.75, 301.25, 303.75, 306.25, 308.75, 311.25, 313.75, 316.25, 318.75, 321.25, 323.75, 326.25, 328.75, 331.25, 333.75, 336.25, 338.75, 341.25, 343.75, 346.25, 348.75, 351.25, 353.75, 356.25])
- time(time)object2000-01-15 00:00:00 ... 2000-12-...
- long_name :
- time
- bounds :
- time_bnds
array([cftime.DatetimeNoLeap(2000, 1, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(2000, 2, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(2000, 3, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(2000, 4, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(2000, 5, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(2000, 6, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(2000, 7, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(2000, 8, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(2000, 9, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(2000, 10, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(2000, 11, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(2000, 12, 15, 0, 0, 0, 0)], dtype=object)
- P0()float64...
- long_name :
- reference pressure
- units :
- Pa
array(100000.)
- date(time)int32...
- long_name :
- date
- format :
- YYYYMMDD
array([20000115, 20000215, 20000315, 20000415, 20000515, 20000615, 20000715, 20000815, 20000915, 20001015, 20001115, 20001215], dtype=int32)
- datesec(time)int32...
- long_name :
- current seconds of current date
array([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0], dtype=int32)
- gw(lat)float64...
- long_name :
- gauss weights
array([0.000137, 0.001093, 0.002185, 0.003275, 0.004361, 0.005443, 0.006518, 0.007587, 0.008647, 0.009697, 0.010737, 0.011765, 0.012781, 0.013782, 0.014768, 0.015739, 0.016692, 0.017626, 0.018542, 0.019437, 0.020311, 0.021162, 0.021991, 0.022795, 0.023575, 0.024329, 0.025056, 0.025756, 0.026427, 0.02707 , 0.027683, 0.028266, 0.028818, 0.029339, 0.029827, 0.030283, 0.030705, 0.031094, 0.031449, 0.03177 , 0.032056, 0.032307, 0.032522, 0.032702, 0.032847, 0.032955, 0.033027, 0.033063, 0.033063, 0.033027, 0.032955, 0.032847, 0.032702, 0.032522, 0.032307, 0.032056, 0.03177 , 0.031449, 0.031094, 0.030705, 0.030283, 0.029827, 0.029339, 0.028818, 0.028266, 0.027683, 0.02707 , 0.026427, 0.025756, 0.025056, 0.024329, 0.023575, 0.022795, 0.021991, 0.021162, 0.020311, 0.019437, 0.018542, 0.017626, 0.016692, 0.015739, 0.014768, 0.013782, 0.012781, 0.011765, 0.010737, 0.009697, 0.008647, 0.007587, 0.006518, 0.005443, 0.004361, 0.003275, 0.002185, 0.001093, 0.000137])
- hyai(ilev)float64...
- long_name :
- hybrid A coefficient at layer interfaces
array([0.002194, 0.004895, 0.009882, 0.018052, 0.029837, 0.044623, 0.061606, 0.078512, 0.077313, 0.075901, 0.074241, 0.072287, 0.069989, 0.067286, 0.064105, 0.060363, 0.055961, 0.050782, 0.04469 , 0.037522, 0.029089, 0.020847, 0.013344, 0.007085, 0.002521, 0. , 0. ])
- hyam(lev)float64...
- long_name :
- hybrid A coefficient at layer midpoints
array([0.003545, 0.007389, 0.013967, 0.023945, 0.03723 , 0.053115, 0.070059, 0.077913, 0.076607, 0.075071, 0.073264, 0.071138, 0.068638, 0.065695, 0.062234, 0.058162, 0.053372, 0.047736, 0.041106, 0.033306, 0.024968, 0.017096, 0.010215, 0.004803, 0.001261, 0. ])
- hybi(ilev)float64...
- long_name :
- hybrid B coefficient at layer interfaces
array([0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.015053, 0.032762, 0.053596, 0.078106, 0.106941, 0.140864, 0.180772, 0.227722, 0.282956, 0.347936, 0.424382, 0.514317, 0.62012 , 0.723536, 0.817677, 0.896215, 0.953476, 0.985112, 1. ])
- hybm(lev)float64...
- long_name :
- hybrid B coefficient at layer midpoints
array([0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.007527, 0.023908, 0.043179, 0.065851, 0.092524, 0.123902, 0.160818, 0.204247, 0.255339, 0.315446, 0.386159, 0.46935 , 0.567219, 0.671828, 0.770606, 0.856946, 0.924846, 0.969294, 0.992556])
- w_stag(slat)float64...
- long_name :
- staggered latitude weights
array([0.000547, 0.00164 , 0.002731, 0.003819, 0.004903, 0.005981, 0.007053, 0.008118, 0.009173, 0.010219, 0.011253, 0.012275, 0.013283, 0.014277, 0.015256, 0.016217, 0.017161, 0.018086, 0.018992, 0.019876, 0.020739, 0.02158 , 0.022396, 0.023188, 0.023955, 0.024696, 0.025409, 0.026095, 0.026752, 0.027381, 0.027979, 0.028546, 0.029082, 0.029587, 0.030059, 0.030498, 0.030904, 0.031276, 0.031614, 0.031917, 0.032186, 0.032419, 0.032617, 0.032779, 0.032905, 0.032996, 0.03305 , 0.033068, 0.03305 , 0.032996, 0.032905, 0.032779, 0.032617, 0.032419, 0.032186, 0.031917, 0.031614, 0.031276, 0.030904, 0.030498, 0.030059, 0.029587, 0.029082, 0.028546, 0.027979, 0.027381, 0.026752, 0.026095, 0.025409, 0.024696, 0.023955, 0.023188, 0.022396, 0.02158 , 0.020739, 0.019876, 0.018992, 0.018086, 0.017161, 0.016217, 0.015256, 0.014277, 0.013283, 0.012275, 0.011253, 0.010219, 0.009173, 0.008118, 0.007053, 0.005981, 0.004903, 0.003819, 0.002731, 0.00164 , 0.000547])
- O3(time, lev, lat, lon)float32...
- units :
- mol/mol
- long_name :
- O3 concentration
- cell_method :
- time: mean
[4313088 values with dtype=float32]
- PS(time, lat, lon)float32...
- units :
- Pa
- long_name :
- Surface pressure
- cell_method :
- time: mean
[165888 values with dtype=float32]
- Conventions :
- CF-1.0
- source :
- CAM
- case :
- ar5_cam_1850-2000_03
- title :
- logname :
- lamar
- host :
- be0207en.ucar.ed
- Version :
- $Name$
- revision_Id :
- $Id$
- initial_file :
- /ptmp/lamar/cam-inputs/ar5_cam_1850_06.cam2.i.1860-01-01-00000.nc
- topography_file :
- /fs/cgd/csm/inputdata/atm/cam/topo/USGS-gtopo30_1.9x2.5_remap_c050602.nc
- history :
- Wed Jan 5 14:56:28 2011: ncks -d time,192,203 /fs/cgd/csm/inputdata/atm/cam/ozone/ozone_1.9x2.5_L26_1850-2005_c091112.nc ozone_1.9x2.5_L26_2000clim_c091112.nc Tue Nov 17 09:59:20 2009: ncks --append -v date,time date_time_1849-2006_c091112.nc ozone_1.9x2.5_L26_1850-2005_c091112.nc Tue Nov 17 09:54:06 2009: ncrcat ozone_1850-1859.r4.nc ozone_1850-1859.r4.nc ozone_1860-1869.r4.nc ozone_1870-1879.r4.nc ozone_1880-1889.r4.nc ozone_1890-1899.r4.nc ozone_1900-1909.r4.nc ozone_1910-1919.r4.nc ozone_1920-1929.r4.nc ozone_1930-1939.r4.nc ozone_1940-1949.r4.nc ozone_1950-1959.r4.nc ozone_1960-1969.r4.nc ozone_1970-1979.r4.nc ozone_1980-1989.r4.nc ozone_1990-1999.r4.nc ozone_2000-2009.r4.nc ozone_2000-2009.r4.nc ozone_1.9x2.5_L26_1850-2005_c091112.nc.672194 11/17/09 09:53:33 eaton:be1105en.ucar.ed:interpic -e T -p 4 -t /fis/cgd/cseg/csm/inputdata/atm/cam/inic/fv/cami_0000-01-01_1.9x2.5_L26_c070408.nc /ptmp/lamar/IPCC_AR5/Climatologies_20091110/ozone_1850-1859.nc ozone_1850-1859.r4.nc
- nco_openmp_thread_number :
- 1
- NCO :
- 4.0.5
- DODS_EXTRA.Unlimited_Dimension :
- time
The pressure levels in this dataset are:
ozone.lev
<xarray.DataArray 'lev' (lev: 26)> array([ 3.544638, 7.388814, 13.967214, 23.944625, 37.23029 , 53.114605, 70.05915 , 85.439115, 100.514695, 118.250335, 139.115395, 163.66207 , 192.539935, 226.513265, 266.481155, 313.501265, 368.81798 , 433.895225, 510.455255, 600.5242 , 696.79629 , 787.70206 , 867.16076 , 929.648875, 970.55483 , 992.5561 ]) Coordinates: * lev (lev) float64 3.545 7.389 13.97 23.94 ... 867.2 929.6 970.6 992.6 Attributes: long_name: hybrid level at midpoints (1000*(A+B)) units: level positive: down standard_name: atmosphere_hybrid_sigma_pressure_coordinate formula_terms: a: hyam b: hybm p0: P0 ps: PS
- lev: 26
- 3.545 7.389 13.97 23.94 37.23 53.11 ... 787.7 867.2 929.6 970.6 992.6
array([ 3.544638, 7.388814, 13.967214, 23.944625, 37.23029 , 53.114605, 70.05915 , 85.439115, 100.514695, 118.250335, 139.115395, 163.66207 , 192.539935, 226.513265, 266.481155, 313.501265, 368.81798 , 433.895225, 510.455255, 600.5242 , 696.79629 , 787.70206 , 867.16076 , 929.648875, 970.55483 , 992.5561 ])
- lev(lev)float643.545 7.389 13.97 ... 970.6 992.6
- long_name :
- hybrid level at midpoints (1000*(A+B))
- units :
- level
- positive :
- down
- standard_name :
- atmosphere_hybrid_sigma_pressure_coordinate
- formula_terms :
- a: hyam b: hybm p0: P0 ps: PS
array([ 3.544638, 7.388814, 13.967214, 23.944625, 37.23029 , 53.114605, 70.05915 , 85.439115, 100.514695, 118.250335, 139.115395, 163.66207 , 192.539935, 226.513265, 266.481155, 313.501265, 368.81798 , 433.895225, 510.455255, 600.5242 , 696.79629 , 787.70206 , 867.16076 , 929.648875, 970.55483 , 992.5561 ])
- long_name :
- hybrid level at midpoints (1000*(A+B))
- units :
- level
- positive :
- down
- standard_name :
- atmosphere_hybrid_sigma_pressure_coordinate
- formula_terms :
- a: hyam b: hybm p0: P0 ps: PS
Take the global average of the ozone climatology, and plot it as a function of pressure (or height)#
# Take global, annual average and convert to Kelvin
weight_ozone = cos(deg2rad(ozone.lat)) / cos(deg2rad(ozone.lat)).mean(dim='lat')
O3_global = (ozone.O3 * weight_ozone).mean(dim=('lat','lon','time'))
O3_global
<xarray.DataArray (lev: 26)> array([7.82792878e-06, 8.64150529e-06, 7.58940028e-06, 5.24567145e-06, 3.17761574e-06, 1.82320006e-06, 9.80756960e-07, 6.22870516e-07, 4.47620550e-07, 3.34481169e-07, 2.62570302e-07, 2.07898125e-07, 1.57074555e-07, 1.12425545e-07, 8.06004999e-08, 6.27826498e-08, 5.42990561e-08, 4.99506089e-08, 4.60075681e-08, 4.22977789e-08, 3.80559071e-08, 3.38768568e-08, 3.12171619e-08, 2.97807119e-08, 2.87980968e-08, 2.75429934e-08]) Coordinates: * lev (lev) float64 3.545 7.389 13.97 23.94 ... 867.2 929.6 970.6 992.6
- lev: 26
- 7.828e-06 8.642e-06 7.589e-06 ... 2.978e-08 2.88e-08 2.754e-08
array([7.82792878e-06, 8.64150529e-06, 7.58940028e-06, 5.24567145e-06, 3.17761574e-06, 1.82320006e-06, 9.80756960e-07, 6.22870516e-07, 4.47620550e-07, 3.34481169e-07, 2.62570302e-07, 2.07898125e-07, 1.57074555e-07, 1.12425545e-07, 8.06004999e-08, 6.27826498e-08, 5.42990561e-08, 4.99506089e-08, 4.60075681e-08, 4.22977789e-08, 3.80559071e-08, 3.38768568e-08, 3.12171619e-08, 2.97807119e-08, 2.87980968e-08, 2.75429934e-08])
- lev(lev)float643.545 7.389 13.97 ... 970.6 992.6
- long_name :
- hybrid level at midpoints (1000*(A+B))
- units :
- level
- positive :
- down
- standard_name :
- atmosphere_hybrid_sigma_pressure_coordinate
- formula_terms :
- a: hyam b: hybm p0: P0 ps: PS
array([ 3.544638, 7.388814, 13.967214, 23.944625, 37.23029 , 53.114605, 70.05915 , 85.439115, 100.514695, 118.250335, 139.115395, 163.66207 , 192.539935, 226.513265, 266.481155, 313.501265, 368.81798 , 433.895225, 510.455255, 600.5242 , 696.79629 , 787.70206 , 867.16076 , 929.648875, 970.55483 , 992.5561 ])
ax = plt.figure(figsize=(10,8)).add_subplot(111)
ax.plot( O3_global * 1.E6, -np.log(ozone.lev/climlab.constants.ps) )
ax.set_xlabel('Ozone (ppm)', fontsize=16)
ax.set_ylabel('Pressure (hPa)', fontsize=16 )
yticks = np.array([1000., 750., 500., 250., 100., 50., 20., 10., 5.])
ax.set_yticks(-np.log(yticks/1000.))
ax.set_yticklabels(yticks)
ax.grid()
ax.set_title('Global, annual mean ozone concentration', fontsize = 24);
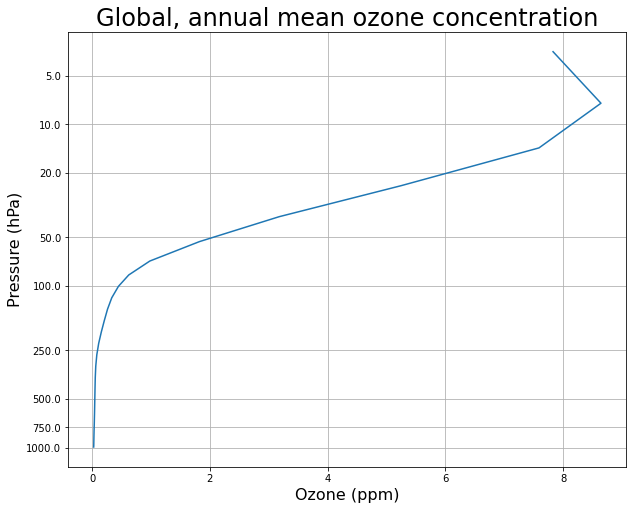
This shows that most of the ozone is indeed in the stratosphere, and peaks near the top of the stratosphere.
Now create a new column model object on the same pressure levels as the ozone data. We are also going set an adjusted lapse rate of 6 K / km.
# the RadiativeConvectiveModel is pre-defined in climlab
# It contains the same components are our previous model
# But here we are specifying a different set of vertical levels.
oz_col = climlab.RadiativeConvectiveModel(lev = ozone.lev, adj_lapse_rate=6)
print(oz_col)
climlab Process of type <class 'climlab.model.column.RadiativeConvectiveModel'>.
State variables and domain shapes:
Ts: (1,)
Tatm: (26,)
The subprocess tree:
Untitled: <class 'climlab.model.column.RadiativeConvectiveModel'>
LW: <class 'climlab.radiation.greygas.GreyGas'>
SW: <class 'climlab.radiation.greygas.GreyGasSW'>
insolation: <class 'climlab.radiation.insolation.FixedInsolation'>
convective adjustment: <class 'climlab.convection.convadj.ConvectiveAdjustment'>
Now we will do something new: let the column absorb some shortwave radiation. We will assume that the shortwave absorptivity is proportional to the ozone concentration we plotted above.
Now we need to weight the absorptivity by the pressure (mass) of each layer.
# This number is an arbitrary parameter that scales how absorptive we are making the ozone
# in our grey gas model
ozonefactor = 75
dp = oz_col.Tatm.domain.lev.delta
epsSW = O3_global.values * dp * ozonefactor
We want to use the field epsSW
as the absorptivity for our SW radiation model.
Let’s see what the absorptivity is current set to:
print(oz_col.subprocess['SW'].absorptivity)
[0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0.
0. 0.]
It defaults to zero.
Before changing this (putting in the ozone), let’s take a look at the shortwave absorption in the column:
oz_col.compute_diagnostics()
oz_col.diagnostics['SW_absorbed_atm']
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.,
0., 0., 0., 0., 0., 0., 0., 0., 0.])
Let’s now put in the ozone:
oz_col.subprocess['SW'].absorptivity = epsSW
print(oz_col.subprocess['SW'].absorptivity)
[3.20948549e-03 3.37750296e-03 4.71182551e-03 4.57614201e-03
3.47591203e-03 2.24450924e-03 1.18884331e-03 7.11369788e-04
5.50761612e-04 4.84170273e-04 4.47141487e-04 4.16507314e-04
3.70212131e-04 3.11733073e-04 2.62922861e-04 2.40936639e-04
2.45147939e-04 2.65307555e-04 2.87482272e-04 2.95567946e-04
2.67120872e-04 2.16427978e-04 1.66169127e-04 1.15468088e-04
6.79353134e-05 3.81013280e-05]
Let’s check how this changes the SW absorption:
oz_col.compute_diagnostics()
oz_col.SW_absorbed_atm
array([1.40571621, 1.47671285, 2.05685574, 1.99236565, 1.50916587,
0.97239284, 0.51429089, 0.30750441, 0.23797743, 0.20913838,
0.19309134, 0.17981756, 0.1597929 , 0.13452308, 0.11343944,
0.10393806, 0.10574109, 0.11442201, 0.12396838, 0.12743553,
0.11515129, 0.09328389, 0.07161233, 0.04975714, 0.02927232,
0.01641659])
It is now non-zero, and largest near the top of the column (also top of the array) where the ozone concentration is highest.
Now it’s time to run the model out to radiative-convective equilibrium
oz_col.integrate_years(1.)
Integrating for 365 steps, 365.2422 days, or 1.0 years.
Total elapsed time is 0.9993368783782377 years.
print(oz_col.ASR - oz_col.OLR)
[-0.00396053]
And let’s now see what we got!
result_list.append(oz_col.state)
name_list.append('Radiative-Convective equilibrium with O3')
# Make a plot to compare observations, Radiative Equilibrium, Radiative-Convective Equilibrium, and RCE with ozone!
plot_soundings(result_list, name_list)
<AxesSubplot:xlabel='Temperature (K)', ylabel='Pressure (hPa)'>
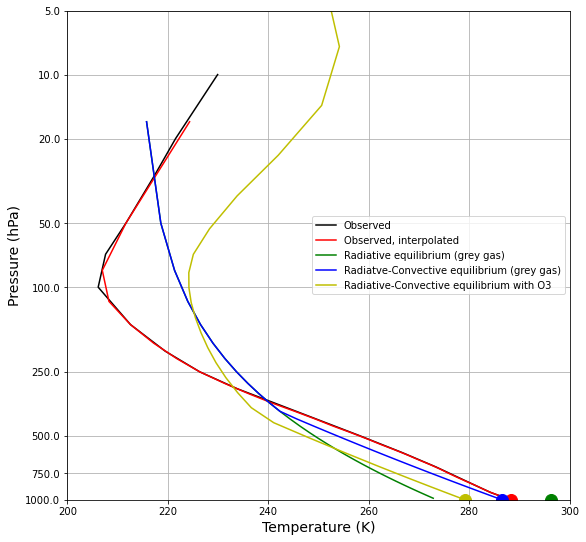
And we finally have something that looks looks like the tropopause, with temperature increasing above at approximately the correct rate.
There are still plenty of discrepancies between this model solution and the observations, including:
Tropopause temperature is too warm, by about 15 degrees.
Surface temperature is too cold
There are a number of parameters we might adjust if we wanted to improve the fit, including:
Longwave absorptivity
Surface albedo
Feel free to experiment! (That’s what models are for, after all).
The take home message#
The dominant effect of stratospheric ozone is to vastly increase the radiative equilibrium temperature in the ozone layer. The temperature needs to be higher so that the longwave emission can balance the shortwave absorption.
Without ozone to absorb incoming solar radiation, the temperature does not increase with height.
This simple grey-gas model illustrates this principle very clearly.
Credits#
This notebook is part of The Climate Laboratory, an open-source textbook developed and maintained by Brian E. J. Rose, University at Albany.
It is licensed for free and open consumption under the Creative Commons Attribution 4.0 International (CC BY 4.0) license.
Development of these notes and the climlab software is partially supported by the National Science Foundation under award AGS-1455071 to Brian Rose. Any opinions, findings, conclusions or recommendations expressed here are mine and do not necessarily reflect the views of the National Science Foundation.